Creative Uses of IDisposable
January 30, 2016
We all know IDisposable interface in NET Framework. It is used to signal to any object with dependencies to other objects when should it dispose of them. IDisposable is a very handy feature together with the using statement, when IDisposable is called automatically when we exit a using code block.
I was reading the Introduction to Rx by Lee Campbell, in preparation of my session for DotNetSpain conference in February. There, I’ve found some creative uses of IDisposable.
Basically, it leverages the fact that Dispose method is called after “using” statement code block exits. So, we can use it to do some wrapping around that code block.
Before the code in the block is executed, the IDisposable constructor will be invoked. After the code is executed, the Dispose method will be invoked. In effect, our code will be surrounded by “Pre” and “Post” actions in an elegant way.
In Campbell’s exemple, a console app can leverage IDisposable to print text in different color inside a code block.
The “wrapper” is a short class named ConsoleColor that implements IDisposable. It remembers the previous console foreground color by saving it in the constructor and restoring it in the Dispose method.
public class ConsoleColor : IDisposable
{
private readonly System.ConsoleColor \_previousColor;
public ConsoleColor(System.ConsoleColor color)
{
\_previousColor = Console.ForegroundColor;
Console.ForegroundColor = color;
}
public void Dispose()
{
Console.ForegroundColor = \_previousColor;
}
}
The calling code just decorates Console.WriteLine code blocks with custom ConsoleColor instances, and voilà, we have a multi-color console application.
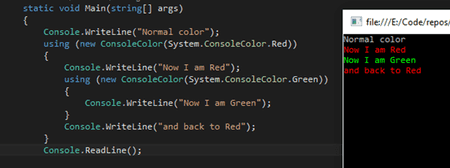